So far, we have only a skeleton of a Django application. We need to create application or app to bring this skeleton to live. To create an app, we have to write command as below:
$ python manage.py startapp home
“home” is the name of our new app, we could name it whatever we want. However, in our multimedia project, we named it “home” because we will use this app to display multimedia item on the home page or the root of the project.
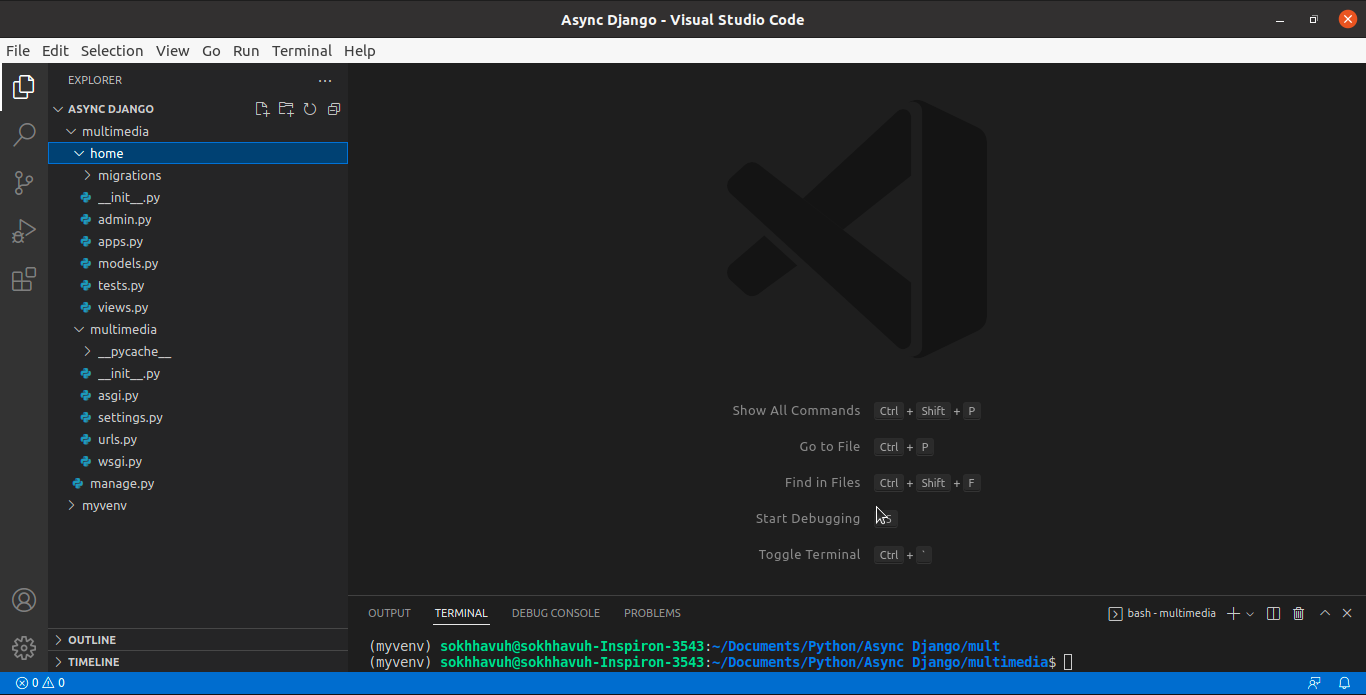
We should notice that when an app is created, a number of necessary files for the app were also created for us to modify them to fit our need. As the result, we are going to modify the file “views.py” to display information we want.
#home/views.py
from django.shortcuts import render
# Create your views here.
def index(request):
kdict = {
'siteTitle':'Khmer Web'
}
return render(request,'index.html',context={'data':kdict})
In Django term, the word “views” means 'controllers" in other web framework. So, what we just did here is to prepare a controller to display some information for the home page on the browser.
On the other hand, the render method in Django does the same job as render method in other web framework - it needs the name of the template to render and a dictionary object as data to insert into the template. We will study in detail about the template language in Django.
Next, we have to define a route linking to the above view by creating a new urls.py file in home folder.
#home/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.index),
]
We have to keep in mind that the above route is only meaningful to the home application, not the whole project. So, we need to let the whole project know about the route to this view by including this local route to global route in the project's urls.py in multimedia folder.
#multimedia/urls.py
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('', include('home.urls')),
path('admin/', admin.site.urls),
]
As we just modified the views.py in home application to render index.html file, we need to create a folder under the name “templates” in home folder in order to store index.html file inside. And this index.html file should look like this:
<!--views/index.html-->
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8"/>
<meta name="viewport" content="width=device-width, initial-scale=1.0"/>
<title>{{ data.siteTitle }}</title>
</head>
<body>
<p>Welcome to Khmer Web Django!</p>
</body>
</html>
The last step is to let the whole project knows about our new app by including its name in settings.py as below:
#multimedia/settings.py
#Application definition
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'home',
]
If we run Django server by writing “python manage.py runserver” on Terminal window and open the browser at the address “http://localhost:8000”, we will see the message “Welcome to Khmer Web Django!” written on the browser.
Mission accomplished!!