Multiple inheritance គឺជាការបង្កើតថ្នាក់មួយបន្តចេញពីថ្នាក់មួយចំនួនទៀត ក្នុងពេលតែមួយ។ ការបង្កើត multiple inheritance ត្រូវធ្វើឡើងដូចខាងក្រោមនេះ៖
class Area():
pi = 3.14
def __init__(self, *dimension):
self.dimension = dimension
def surface(self):
return self.dimension
class Volume():
pi = 3.1415
def __init__(self, *dimension):
self.dimension = dimension
def volume(self):
return self.dimension
class Cube(Area, Volume):
pi = 3.141592
def __init__(self, *dimension):
self.dimension = dimension
def surface(self):
s = self.dimension[0] * self.dimension[1] * 6
print("The cube's surface is", s)
def volume(self):
v = self.dimension[0] * self.dimension[1] * self.dimension[2]
print("The cube's volume is", v)
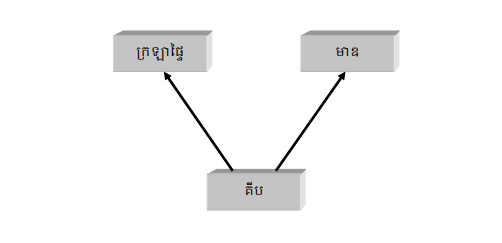
ក្នុងករណីមានការបង្កើត multiple inheritance, នៅពេលដែល attribute ផ្សេងៗត្រូវយកទៅប្រើ ការស្វែងរក attribute ទាំងនោះ ត្រូវធ្វើឡើងដូចនៅក្នុងរូបខាងក្រោមនេះ៖
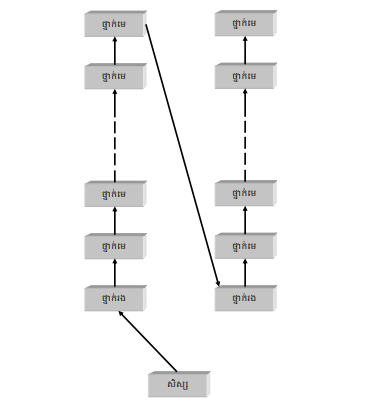
រូបខាងលើនេះបង្ហាញថា បើសិនជាការយក attribute នានាទៅប្រើតាមរយៈ instance នៃ subclass ការស្វែងរកវត្ថុនោះត្រូវធ្វើឡើងនៅក្នុង instance នោះមុន រួចបានឡើងទៅថ្នាក់របស់វា រួចឡើងទៅ superclass របស់វា ជាបន្តបន្ទាប់តាមសញ្ញាព្រួញ រហូតដល់ attribute នោះត្រូវរកឃើញទើបឈប់។ ហើយក្នុងករណីដែលមាន attribute មានឈ្មោះដូចគ្នាជាច្រើននៅក្នុងថ្នាក់ទាំងនោះ attribute ដែលត្រូវយកមកប្រើ គឺជា attribute ដែលត្រូវរកឃើញមុនគេ។ ពិនិត្យកម្មវិធីខាងក្រោមនេះ៖
class Area():
pi = 3.14
def __init__(self, *dimension):
self.dimension = dimension
def surface(self):
return self.dimension
class Volume():
pi = 3.1415
def __init__(self, *dimension):
self.dimension = dimension
def volume(self):
return self.dimension
class Cube(Area, Volume):
pi = 3.141592
def __init__(self, *dimension):
self.dimension = dimension
def surface(self):
s = self.dimension[0] * self.dimension[1] * 6
print("The cube's surface is", s)
def volume(self):
v = self.dimension[0] * self.dimension[1] * self.dimension[2]
print("The cube's volume is", v)
cube = Cube(25, 5, 10)
cube.surface()
cube.volume()
print("The value of pi is", cube.pi)
ចំពោះ overridden method នៅក្នុង superclass បើសិនជាយើងចង់ call method ទាំងនោះនៅក្នុង subclass យើងត្រូវធ្វើដូចខាងក្រោមនេះ៖
class Area():
pi = 3.14
def __init__(self, *dimension):
self.dimension = dimension
def surface(self):
return self.dimension
class Volume():
pi = 3.1415
def __init__(self, *dimension):
self.dimension = dimension
def volume(self):
return self.dimension
class Cube(Area, Volume):
pi = 3.141592
def __init__(self, width, height, depth):
super().__init__(width, height)
Volume.__init__(self, width, height, depth)
def surface(self):
dimension = super().surface()
s = dimension[0] * dimension[1] * 6
print("The cube's surface is", s)
def volume(self):
dimension = super().volume()
v = dimension[0] * dimension[1] * dimension[2]
print("The cube's volume is", v)
cube = Cube(25, 5, 10)
cube.surface()
cube.volume()